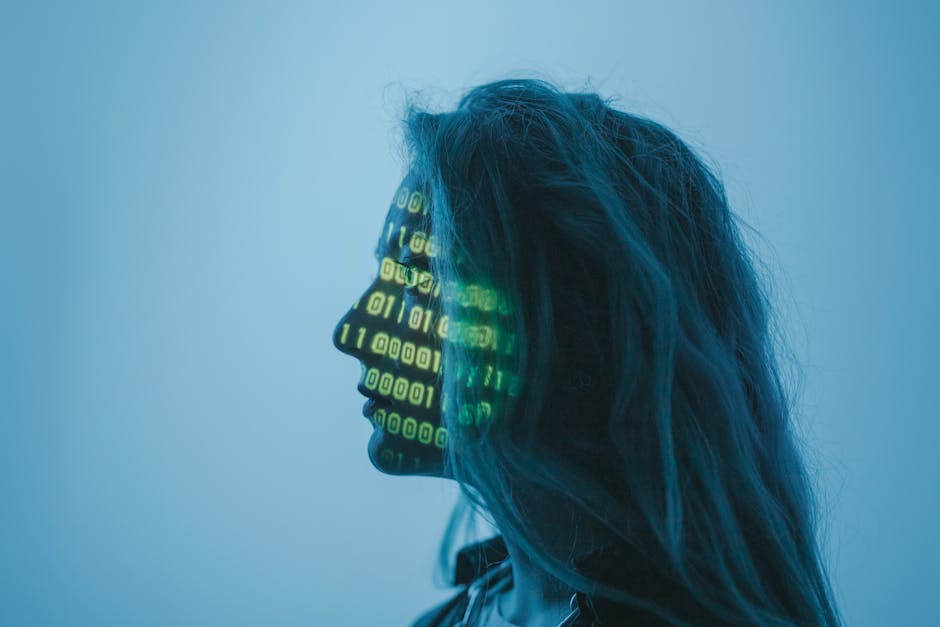
For anyone diving into AI technology, understanding the chatgpt api tutorial is a crucial step. The ChatGPT API is a powerful tool designed by OpenAI, allowing developers to integrate advanced AI capabilities into their own applications seamlessly. This guide will get you started with the basics, taking you all the way from obtaining an OpenAI API key to setting up your first chat and text completions.
Here’s a quick overview of what you need to get started:
- Sign up for an OpenAI account and generate your unique API key.
- Choose the right API model for your needs, such as GPT-3.5-turbo or GPT-4.
- Set up your API calls in a program using the OpenAI library.
- Understand key parameters like role, temperature, and max tokens for response customization.
As a tech-savvy digital marketer, mastering these steps can significantly streamline your content marketing and SEO strategies.
I’m Jeffrey Castillo, known as digitaljeff, and I have been at the forefront of tech advancements for over two decades. My work with content creators has been focused on empowering them with the latest tools, making the chatgpt api tutorial a natural fit in my expertise, driving both engagement and growth.
Glossary for chatgpt api tutorial:
– ChatGPT tutorials
– how to use chatgpt
– openai chatgpt tutorial
Getting Started with ChatGPT API
How to Generate Your API Key
To begin using the ChatGPT API, the first step is to generate your API key. This key acts as your unique identifier when interacting with OpenAI’s powerful models.
-
Sign Up for an OpenAI Account: If you haven’t already, head over to the OpenAI platform and sign up for an account. If you already have an account, simply log in.
-
Access the API Keys Section: Once logged in, steer to the API keys section in your account settings.
-
Create a New Secret Key: Click on the Create new secret key button. This will generate a new API key for you. This is the only time you’ll be able to view the full key, so make sure to copy it and store it in a secure place.
-
Save Your API Key: Since you can’t view the key again, save it in a secure location like a password manager or an encrypted file.
By following these steps, you ensure that you have the necessary credentials to access the ChatGPT API.
Understanding API Models and Pricing
Once you have your API key, the next step is to understand the different API models and how pricing works.
-
API Models: OpenAI offers several models, including GPT-3.5-turbo and GPT-4. Each model has its own strengths. GPT-3.5-turbo is known for being cost-effective and suitable for most applications, while GPT-4 offers improved capabilities and performance.
-
Token Usage: The API operates on a token-based system. Tokens are chunks of text, and both input and output text consume tokens. Understanding how many tokens your application uses is crucial in estimating costs.
-
Pricing Details: While specific pricing information isn’t provided here, costs generally depend on the model chosen and the number of tokens processed. It’s advisable to monitor your token usage through the OpenAI platform to manage expenses effectively.
By understanding these aspects, you’ll be better prepared to make informed decisions about which model to use and how to manage your API usage efficiently.
This foundational knowledge sets you up to dive deeper into using the ChatGPT API for both chat and text completions.
Using the ChatGPT API
ChatGPT API Tutorial: Basic Setup
Getting started with the ChatGPT API involves setting up your environment to make API calls. We’ll walk through the basic setup using Python, which is a popular choice due to its simplicity and power.
1. Install the OpenAI Python Library
First, ensure you have Python installed on your system. Then, install the OpenAI library using pip:
bash
pip install openai
2. Configure Your API Key
Once installed, you’ll need to configure your API key, which you generated earlier. This key allows your application to communicate with OpenAI’s servers.
“`python
import openai
openai.api_key = “YOUR_API_KEY”
“`
Replace "YOUR_API_KEY"
with your actual API key.
3. Making Your First API Call
Here’s a basic example of how to make an API call for chat completion:
“`python
completion = openai.ChatCompletion.create(
model=”gpt-3.5-turbo”,
temperature=0.8,
max_tokens=150,
messages=[
{“role”: “system”, “content”: “You are a helpful assistant.”},
{“role”: “user”, “content”: “What’s the weather like today?”}
]
)
print(completion.choices[0].message.content)
“`
This script sets up a conversation where the system defines the assistant’s role, and the user asks a question. The model responds based on the context provided.
ChatGPT API for Chat Completion
When using the ChatGPT API for chat completion, understanding the role parameter is crucial. The roles include:
- System: Sets the behavior of the assistant. For example, “You are a helpful assistant.”
- User: Represents input from the user.
- Assistant: Contains responses generated by the model.
Temperature and Max Tokens
- Temperature: Controls randomness. A higher value like 0.8 makes output more varied, while lower values make it more focused.
- Max Tokens: Limits the length of the response. Ensure it’s set according to your application’s needs.
ChatGPT API for Text Completion
For text completion, the setup is similar but focuses on completing given text inputs. Here’s an example:
“`python
completion = openai.ChatCompletion.create(
model=”gpt-3.5-turbo”,
temperature=0.5,
max_tokens=100,
messages=[
{“role”: “user”, “content”: “Once upon a time in a land far away,”}
]
)
print(completion.choices[0].message.content)
“`
This call asks the model to continue the given text prompt. Adjust the temperature and max tokens to fit the desired output style and length.
Understanding the Response Format
The API’s response includes several key components:
json
{
"choices": [
{
"message": {
"content": "Response from the model",
"role": "assistant"
},
"finish_reason": "stop",
"index": 0
}
],
"usage": {
"completion_tokens": 50,
"prompt_tokens": 10,
"total_tokens": 60
}
}
- Message Content: The main response from the model.
- Finish Reason: Indicates why the response ended (e.g., “stop”).
- Token Usage: Provides details on how many tokens were used, helping manage costs.
By following these steps and understanding these components, you can effectively use the ChatGPT API for a variety of applications.
Next, we’ll explore how to build applications using the ChatGPT API, focusing on API endpoints and practical coding examples.
Building Applications with ChatGPT API
Using API Endpoints in Your Code
When building applications with the ChatGPT API, understanding how to use API endpoints is crucial. The endpoint is the URL to which your application sends requests to interact with the API. For ChatGPT, this involves sending a payload containing your query and receiving a response.
Here’s how to set up your API endpoint:
- Install Necessary Libraries
Before you start, ensure that you have the requests
library installed, which allows your Python application to send HTTP requests easily.
bash
pip install requests
- Configure Your API Key and Endpoint
Your API key is your access pass to OpenAI’s services. Keep it secure and never share it publicly.
“`python
import requests
API_KEY = “YOUR_API_KEY”
API_ENDPOINT = “https://api.openai.com/v1/chat/completions”
“`
- Prepare Your Request
Set up the headers and payload for the request. Headers generally include your authorization details, while the payload contains the data you want to send.
“`python
headers = {
“Content-Type”: “application/json”,
“Authorization”: f”Bearer {API_KEY}”
}
payload = {
“model”: “gpt-3.5-turbo”,
“messages”: [
{“role”: “system”, “content”: “You are a helpful assistant.”},
{“role”: “user”, “content”: “Tell me a joke.”}
],
“temperature”: 0.7,
“max_tokens”: 150
}
“`
- Make the API Call
Use the requests
library to send the request and handle the response.
“`python
response = requests.post(API_ENDPOINT, headers=headers, json=payload)
if response.status_code == 200:
print(response.json()[‘choices’][0][‘message’][‘content’])
else:
print(f”Error: {response.status_code}”)
“`
This code sends a request to the API and prints the response content if successful. Adjust the payload and headers as needed for your specific application.
Creating Programs with OpenAI Library
The OpenAI library offers a more streamlined approach to interacting with the API. It abstracts some of the complexities involved in sending HTTP requests directly.
Steps to create programs using the OpenAI library:
- Install the OpenAI Library
Ensure you have the library installed:
bash
pip install openai
- Set Up the API Key
Configure your API key in your script:
“`python
import openai
openai.api_key = “YOUR_API_KEY”
“`
- Write Your Application
Here’s an example of a simple program using the OpenAI library to get a chat completion:
“`python
response = openai.ChatCompletion.create(
model=”gpt-3.5-turbo”,
temperature=0.6,
max_tokens=150,
messages=[
{“role”: “system”, “content”: “You are a creative writer.”},
{“role”: “user”, “content”: “Write a short story about a dragon.”}
]
)
print(response.choices[0].message.content)
“`
This script sets up a creative writing assistant and asks for a short story, demonstrating how you can quickly integrate ChatGPT into your applications.
Best Practices:
- Secure Your API Key: Always keep your API key hidden, especially in public repositories.
- Optimize Token Usage: Monitor token usage to manage costs effectively.
- Handle Errors Gracefully: Implement error handling to manage API call failures smoothly.
- Experiment with Parameters: Adjust parameters like
temperature
andmax_tokens
to fit your application’s needs.
By following these steps and best practices, you can build powerful applications using the ChatGPT API, enhancing user experiences with AI-driven interactions.
Next, let’s explore some practical applications and examples of how the ChatGPT API can be leveraged in real-world scenarios.
Conclusion
The ChatGPT API offers immense potential for developers looking to improve their applications with AI-driven capabilities. As we’ve explored throughout this guide, integrating this technology can transform simple apps into powerful tools that engage users with intelligent and dynamic interactions.
ChatGPT API Potential
The versatility of the ChatGPT API is one of its greatest strengths. Whether you’re developing a chatbot that tells jokes or a sophisticated tool for content generation, the API can adapt to various use cases. The ability to process natural language inputs and provide coherent, context-aware responses makes it an invaluable asset for applications across industries.
Best Practices
To maximize the benefits of using the ChatGPT API, consider these best practices:
-
Secure Your API Key: Protect your API key by storing it securely and avoiding exposure in public code repositories. This prevents unauthorized access and potential misuse.
-
Optimize Token Usage: Keep an eye on token consumption to manage costs effectively. Understanding the token limits of different models, like GPT-3.5-turbo and GPT-4, can help you optimize usage.
-
Experiment with Parameters: Adjusting parameters like
temperature
andmax_tokens
can significantly impact the quality of responses. Experiment with these settings to achieve the desired balance between creativity and precision. -
Implement Error Handling: Ensure your application gracefully handles API call failures by implementing robust error handling mechanisms. This improves reliability and user experience.
Unsigned Creator Community
At Unsigned Creator Community, we are committed to empowering creators with certified AI tools and resources. Our focus is on providing top-tier solutions for content marketing and SEO, helping online creators and agencies achieve their goals. By leveraging the ChatGPT API, you can improve your digital strategy and open up new opportunities for innovation.
For more insights and resources on integrating AI into your projects, explore our AI solutions page.
Final Thoughts
As AI continues to evolve, the possibilities for its application in real-world scenarios are endless. The ChatGPT API is just the beginning. By integrating this technology wisely and following best practices, developers can create applications that not only meet but exceed user expectations. Accept the potential of AI, and let it drive your projects to new heights.